ipython#
Motivation#
The classical development cycle is sometimes caricatured as Edit-Run-Debug loop. Some debugging tasks are greatly simplified if one can quickly run code snippets and immediately see the results. This is the starting point for “interactive programming” that enables exploratory coding. The Python interpreter offers a built-in Read-Eval-Print-Loop (REPL) shell.
$ python
Python 3.11.2
>>> 1+1
2
>>> exit()
However the default shell has limited functionality. Therefore, back in 2001, a PhD student in particle physics started developing Interactive Python (IPython). The same project later evolved into ipython notebook and finally Jupyter.
Usage#
Interactive shell#
The IPython shell offers many features
object introspection
multi-line editing and tab completion
numbered input/output prompts with command history
rich output of data structures with syntax highlighting
magic commands and access to shell commands
Invoke the ipython
terminal command to get started.
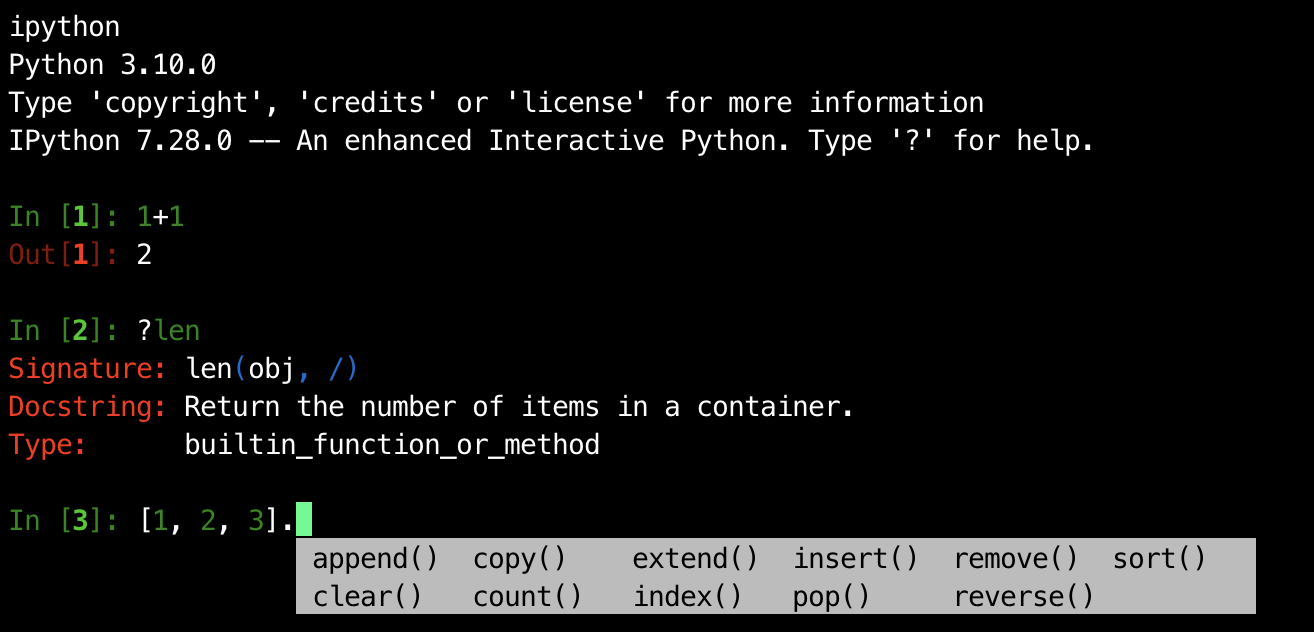
Magic commands#
IPython has so-called magic commands to enhance the interactive shell with additional features. Line magics are prefixed with a single %
, cell magics with %%
and commands starting with !
are shell magics. These commands also work inside Jupyter notebooks.
%quickref # show quick reference of magic commands
%magic # general information about magic commands
%aimport pkg # import package and automatically reload on code changes
%pinfo obj # print info about object. Same as obj? or ?obj
%pinfo2 obj # print more info about object. Same as obj?? or ??obj
%pdoc obj # print docstring of object
%psource obj # print source code of object definition
%pfile obj # print file containing object definition
%run file.py # execute code from file
%prun file.py # execute code from file with profiler
%mprun file.py # execute code from file with memory profiler
%lprun file.py # execute code from file with line profiler
%%timeit # time code execution of current cell
%%bash # execute cell contents as bash code (or %%html, %%javascript, etc)
%cd ~/ # change directory
!ls # execute shell command
files = !ls # execute shell command and capture output in Python variable
Attach interactive debugger#
Another useful feature is to start an embedded IPython shell directly from your code. This provides access to all the project’s variables for live inspection and debugging, similar to setting a breakpoint in the IDE.
import IPython
a = 1
IPython.embed()